下面的示例展示了如何为业务对象实现树结构。
在本示例中,自定义 MyData 类支持 IVirtualTreeListData 接口,允许该类的对象在 TreeList 控件中呈现。 此对象实现了接口的所有三个方法:
- VirtualTreeGetChildNodes —— 返回根节点或子节点的列表。
- VirtualTreeGetCellValue —— 返回节点单元格的取值。
- VirtualTreeSetCellValue —— 保存由最终用户对对象作出的更改。
MyData 类实现如下:
C# | ![]() |
---|---|
using DevExpress.XtraTreeList; using DevExpress.XtraTreeList.Columns; // Represents a sample Business Object public class MyData : TreeList.IVirtualTreeListData { protected MyData parentCore; protected ArrayList childrenCore = new ArrayList(); protected object[] cellsCore; public MyData(MyData parent, object[] cells) { // Specifies the parent node for the new node. this.parentCore = parent; // Provides data for the node's cell. this.cellsCore = cells; if (this.parentCore != null) { this.parentCore.childrenCore.Add(this); } } void TreeList.IVirtualTreeListData.VirtualTreeGetChildNodes( VirtualTreeGetChildNodesInfo info) { info.Children = childrenCore; } void TreeList.IVirtualTreeListData.VirtualTreeGetCellValue( VirtualTreeGetCellValueInfo info) { info.CellData = cellsCore[info.Column.AbsoluteIndex]; } void TreeList.IVirtualTreeListData.VirtualTreeSetCellValue(VirtualTreeSetCellValueInfo info) { cellsCore[info.Column.AbsoluteIndex] = info.NewCellData; } } |
Visual Basic | ![]() |
---|---|
Imports DevExpress.XtraTreeList Imports DevExpress.XtraTreeList.Columns ' Represents a sample Business Object. Public Class MyData Implements TreeList.IVirtualTreeListData Protected parentCore As MyData Protected childrenCore As New ArrayList() Protected cellsCore() As Object Public Sub New(ByVal parent As MyData, ByVal cells() As Object) ' Specifies the parent node for the new node. Me.parentCore = parent ' Provides data for the node's cell. Me.cellsCore = cells If Not (Me.parentCore Is Nothing) Then Me.parentCore.childrenCore.Add(Me) End If End Sub Sub VirtualTreeGetChildNodes(ByVal info As VirtualTreeGetChildNodesInfo) _ Implements TreeList.IVirtualTreeListData.VirtualTreeGetChildNodes info.Children = childrenCore End Sub Sub VirtualTreeGetCellValue(ByVal info As VirtualTreeGetCellValueInfo) _ Implements TreeList.IVirtualTreeListData.VirtualTreeGetCellValue info.CellData = cellsCore(info.Column.AbsoluteIndex) End Sub Sub VirtualTreeSetCellValue(ByVal info As VirtualTreeSetCellValueInfo) _ Implements TreeList.IVirtualTreeListData.VirtualTreeSetCellValue cellsCore(info.Column.AbsoluteIndex) = info.NewCellData End Sub End Class |
下列代码创建 MyData 类的一个表示 TreeList 控件数据源的对象,使用节点装载它并绑定到 TreeList。
C# | ![]() |
---|---|
public partial class Form1 : Form { public Form1() { InitializeComponent(); InitData(); } void InitData() { // Represents the root of the tree. MyData tlDataSource = new MyData(null, null); // Represents the first root node. MyData rootNode1 = new MyData(tlDataSource, new string[] { "Project A", "High" }); MyData node1 = new MyData(rootNode1, new string[] { "Deliverable 1", "Normal" }); MyData node2 = new MyData(node1, new string[] { "This task is mine A", "High" }); MyData node3 = new MyData(node1, new string[] { "This task isn't mine A", "Low" }); // Represents the second root node. MyData rootNode2 = new MyData(tlDataSource, new string[] { "Project B", "Normal" }); // Represents the child node of the second root node. MyData node5 = new MyData(rootNode2, new string[] { "This task is mine B", "High" }); treeList1.DataSource = tlDataSource; TreeListColumn col1 = new TreeListColumn(); col1.Caption = "Name"; col1.VisibleIndex = 0; TreeListColumn col2 = new TreeListColumn(); col2.Caption = "Priority"; col2.VisibleIndex = 1; treeList1.Columns.AddRange(new TreeListColumn[] {col1, col2}); } } |
Visual Basic | ![]() |
---|---|
Public Class Form1 Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load InitData() End Sub Sub InitData() ' Represents the root of the tree. Dim tlDataSource = New MyData(Nothing, Nothing) ' Represents the first root node. Dim rootNode1 = New MyData(tlDataSource, New String() {"Project A", "High"}) Dim node1 = New MyData(rootNode1, New String() {"Deliverable 1", "Normal"}) Dim node2 = New MyData(node1, New String() {"This task is mine A", "High"}) Dim node3 = New MyData(node1, New String() {"This task isn't mine A", "Low"}) ' Represents the second root node. Dim rootNode2 = New MyData(tlDataSource, New String() {"Project B", "Normal"}) ' Represents the child node of the second root node. Dim node5 = New MyData(rootNode2, New String() {"This task is mine B", "High"}) TreeList1.DataSource = tlDataSource Dim col1 As TreeListColumn = New TreeListColumn() col1.Caption = "Name" col1.VisibleIndex = 0 Dim col2 As TreeListColumn = New TreeListColumn() col2.Caption = "Priority" col2.VisibleIndex = 1 TreeList1.Columns.AddRange(New TreeListColumn() {col1, col2}) End Sub End Class |
下面的插图展示了结果:
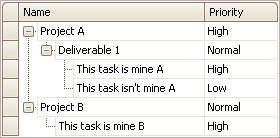