下面的示例通过 TreeList.CustomDrawColumnHeader、TreeList.CustomDrawFooter、TreeList.CustomDrawFooterCell 和 TreeList.CustomDrawNodeIndicator 事件自定义绘制了列标头、汇总脚注、脚注单元格和指示器单元格。
定义了三个实现自定义绘制的方法。 这些方法被用于绘制凸起元素的背景、绘制凹下元素的背景、以及使用预定义的元素内对齐方式绘制文本。
下面的插图展示了运行结果。
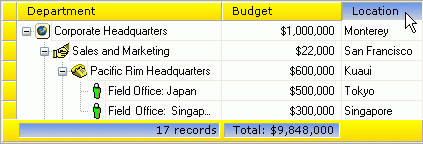
C# | ![]() |
---|---|
using System.Drawing.Drawing2D; using DevExpress.XtraTreeList; //... // custom painting column headers private void treeList1_CustomDrawColumnHeader(object sender, DevExpress.XtraTreeList.CustomDrawColumnHeaderEventArgs e) { if (e.Pressed) paintSunkenBackground(e.Graphics, e.Bounds); else paintRaisedBackground(e.Graphics, e.Bounds); if (e.ColumnType == HitInfoType.Column) paintHAlignedText(e.Graphics, e.Bounds, e.Column.Caption, StringAlignment.Near); e.Handled = true; } // custom painting the summary footer private void treeList1_CustomDrawFooter(object sender, DevExpress.XtraTreeList.CustomDrawEventArgs e) { paintRaisedBackground(e.Graphics, e.Bounds); e.Handled = true; } // custom painting the cells of the summary footer private void treeList1_CustomDrawFooterCell(object sender, DevExpress.XtraTreeList.CustomDrawFooterCellEventArgs e) { if (e.Text == String.Empty) return; paintSunkenBackground(e.Graphics, e.Bounds); paintHAlignedText(e.Graphics, e.Bounds, e.Text, StringAlignment.Far); e.Handled = true; } // custom painting the indicator cells private void treeList1_CustomDrawNodeIndicator(object sender, DevExpress.XtraTreeList.CustomDrawNodeIndicatorEventArgs e) { paintRaisedBackground(e.Graphics, e.Bounds); e.Handled = true; } // Custom draw methods: // paints the background of raised elements private void paintRaisedBackground(Graphics g, Rectangle bounds) { LinearGradientBrush brush = new LinearGradientBrush(bounds, Color.Yellow, Color.Gold, LinearGradientMode.Vertical); g.FillRectangle(brush, bounds); ControlPaint.DrawBorder3D(g, bounds, Border3DStyle.RaisedInner); } // paints the background of sunken elements private void paintSunkenBackground(Graphics g, Rectangle bounds) { LinearGradientBrush brush = new LinearGradientBrush(bounds, Color.CornflowerBlue, Color.LightGray, LinearGradientMode.Vertical); g.FillRectangle(brush, bounds); ControlPaint.DrawBorder3D(g, bounds, Border3DStyle.SunkenOuter); } // paints the aligned text private void paintHAlignedText(Graphics g, Rectangle bounds, string text, StringAlignment align) { SolidBrush textBrush = new SolidBrush(Color.Black); StringFormat outStringFormat = new StringFormat(); outStringFormat.Alignment = align; outStringFormat.LineAlignment = StringAlignment.Center; outStringFormat.FormatFlags = StringFormatFlags.NoWrap; outStringFormat.Trimming = StringTrimming.EllipsisCharacter; g.DrawString(text, new Font("Verdana", 8), textBrush, bounds, outStringFormat); } |
Visual Basic | ![]() |
---|---|
Imports System.Drawing.Drawing2D Imports DevExpress.XtraTreeList '... ' painting column headers in a custom manner Private Sub TreeList1_CustomDrawColumnHeader(ByVal sender As Object, ByVal e As DevExpress.XtraTreeList.CustomDrawColumnHeaderEventArgs) _ Handles TreeList1.CustomDrawColumnHeader If e.Pressed Then PaintSunkenBackground(e.Graphics, e.Bounds) Else PaintRaisedBackground(e.Graphics, e.Bounds) End If If e.ColumnType = HitInfoType.Column Then PaintHAlignedText(e.Graphics, e.Bounds, e.Column.Caption, StringAlignment.Near) End If e.Handled = True End Sub ' custom painting the summary footer Private Sub TreeList1_CustomDrawFooter(ByVal sender As Object, ByVal e As DevExpress.XtraTreeList.CustomDrawEventArgs) _ Handles TreeList1.CustomDrawFooter PaintRaisedBackground(e.Graphics, e.Bounds) e.Handled = True End Sub ' custom painting the cells of the summary footer Private Sub TreeList1_CustomDrawFooterCell(ByVal sender As Object, ByVal e As DevExpress.XtraTreeList.CustomDrawFooterCellEventArgs) _ Handles TreeList1.CustomDrawFooterCell If e.Text = String.Empty Then Exit Sub PaintSunkenBackground(e.Graphics, e.Bounds) PaintHAlignedText(e.Graphics, e.Bounds, e.Text, StringAlignment.Far) e.Handled = True End Sub ' custom painting indicator cells Private Sub TreeList1_CustomDrawNodeIndicator(ByVal sender As Object, ByVal e As DevExpress.XtraTreeList.CustomDrawNodeIndicatorEventArgs) _ Handles TreeList1.CustomDrawNodeIndicator PaintRaisedBackground(e.Graphics, e.Bounds) e.Handled = True End Sub ' Custom draw methods: ' paints the background of raised elements Private Sub PaintRaisedBackground(ByVal g As Graphics, ByVal Bounds As Rectangle) Dim Brush As New LinearGradientBrush(Bounds, Color.Yellow, Color.Gold, LinearGradientMode.Vertical) g.FillRectangle(Brush, Bounds) ControlPaint.DrawBorder3D(g, Bounds, Border3DStyle.RaisedInner) End Sub ' paints the background of sunken elements Private Sub PaintSunkenBackground(ByVal g As Graphics, ByVal Bounds As Rectangle) Dim Brush As New LinearGradientBrush(Bounds, Color.CornflowerBlue, Color.LightGray, LinearGradientMode.Vertical) g.FillRectangle(Brush, Bounds) ControlPaint.DrawBorder3D(g, Bounds, Border3DStyle.SunkenOuter) End Sub ' paints the aligned text Private Sub PaintHAlignedText(ByVal g As Graphics, ByVal Bounds As Rectangle, ByVal Text As String, ByVal Align As StringAlignment) Dim TextBrush As New SolidBrush(Color.Black) Dim OutStringFormat As New StringFormat() OutStringFormat.Alignment = Align OutStringFormat.LineAlignment = StringAlignment.Center OutStringFormat.FormatFlags = StringFormatFlags.NoWrap OutStringFormat.Trimming = StringTrimming.EllipsisCharacter Dim BoundsF As New RectangleF(Bounds.X, Bounds.Y, Bounds.Width, Bounds.Height) g.DrawString(Text, New Font("Verdana", 8), TextBrush, BoundsF, OutStringFormat) End Sub |