弹出式容器编辑器
PopupContainerEdit 编辑器允许在其弹出窗口中显示控件。 可以把任意控件组合放置到一个特殊设计的面板控件中,并且把此面板用作编辑器的弹出窗口。 下面的插图展示了一个示例。
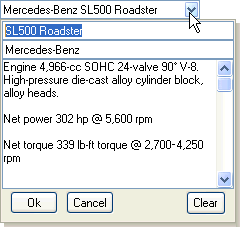
要在弹出式容器编辑器的下拉窗口中显示控件,则需要把控件放置到一个由 PopupContainerControl 控件表示的外部面板中。 然后把此面板指派到编辑器的 RepositoryItemPopupContainerEdit.PopupControl 属性。 一旦指派了面板,则此面板在运行时刻在窗体中不可视,仅作为绑定编辑器的弹出窗口进行显示。
弹出式容器编辑器可以用于编辑包含了非常规数据的数据字段。例如,字段值可能由包含 0 和 1 字符——指明某个特定标记是否被启用——的字符串来表示。 然后可以通过在其下拉窗口中显示了复选框 (每个复选框对应每个选项) 的弹出式容器编辑器控件来编辑该字段的值。 也可以通过把逻辑分组的控件放置到其下拉窗口中,使用弹出式容器编辑器来节省屏幕空间。
通常,需要根据位于弹出窗口内控件的状态来同步编辑器的取值和显示文本。 要更新编辑器的取值和文本,以响应最终用户所作的更改,则接管 RepositoryItemPopupContainerEdit.QueryResultValue 和 RepositoryItemPopupContainerEdit.QueryDisplayText 事件。 当弹出窗口关闭并且未取消所作更改时,这些事件发生。 这样,可以检查控件的状态,并且把适当的值指派到编辑器。 例如,要更新下拉窗口内的控件,可以接管 RepositoryItemPopupBase.QueryPopUp 事件。 在弹出窗口正要打开之前,该事件发生。
示例 —— 在下拉窗口中显示列表框
假设需要在弹出窗口内显示一个列表框控件和 OK、Cancel 按钮。 此列表框允许最终用户选择多个列表项。 按钮用于关闭下拉窗口,并接受或取消所作的修改。
按照上面的规定,应该把这些控件放置到一个 PopupContainerControl 面板中,按顺序将此面板指派到编辑器的 RepositoryItemPopupContainerEdit.PopupControl 属性。
此弹出式容器编辑器的编辑值是一个包含了列表框选中项的数组。 显示文本反映选中项的数目。
窗体的构造函数使用一个空 ArrayList 对象初始化编辑值。
PopupContainerEdit.QueryResultValue 事件处理程序获取选中项,并把它们添加到表示编辑值的数组中。 在下面的代码中,位于弹出窗口内的列表框被称为listBox1。
PopupContainerEdit.QueryDisplayText 事件被接管,以显示选中项的数目,也就是存储编辑值的数组中元素的数目。
OK 和 Cancel 按钮关闭弹出窗口,保存或取消所作的修改。 因此,它们的 Click 事件处理程序分别调用 PopupBaseEdit.ClosePopup 和 PopupBaseEdit.CancelPopup 方法。 通过弹出控件的 PopupContainerControl.OwnerEdit 属性返回编辑器。
最终用户可以修改选中项,然后单击 Cancel 按钮或按下 ESC 键来取消更改。 在这种情况下,从编辑器的编辑值中获取前面的选中项,以恢复选中项。 PopupBaseEdit.CloseUp 事件处理程序执行此任务。
C# | ![]() |
---|---|
using DevExpress.XtraEditors.Controls; public Form1() { InitializeComponent(); //... popupContainerEdit1.EditValue = new ArrayList(); } private void popupContainerEdit1_QueryResultValue(object sender, QueryResultValueEventArgs e) { //Get the list box displayed in the editor's dropdown ListBox listBox = findChildListBox((sender as PopupContainerEdit).Properties.PopupControl); //Add selected items to the array specified by the editor's edit value ArrayList ar = e.Value as ArrayList; ar.Clear(); ar.AddRange(listBox.SelectedItems); } private void popupContainerEdit1_QueryDisplayText(object sender, QueryDisplayTextEventArgs e) { //Return the string representing the selected item count int itemCount = (e.EditValue as ArrayList).Count; e.DisplayText = itemCount.ToString() + " item(s) selected"; } private void buttonOk_Click(object sender, System.EventArgs e) { Control button = sender as Control; //Close the dropdown accepting the user's choice (button.Parent as PopupContainerControl).OwnerEdit.ClosePopup(); } private void buttonCancel_Click(object sender, System.EventArgs e) { Control button = sender as Control; //Close the dropdown discarding the user's choice (button.Parent as PopupContainerControl).OwnerEdit.CancelPopup(); } private void popupContainerEdit1_CloseUp(object sender, CloseUpEventArgs e) { //Restore selected items if the user's choice should be discarded if (! e.AcceptValue) { PopupContainerEdit popupContainerEditor = sender as PopupContainerEdit; //Get the list box displayed in the dropdown of the popup container editor ListBox listBox = findChildListBox(popupContainerEditor.Properties.PopupControl); if (listBox != null) { //Clear existing selection listBox.ClearSelected(); //Restore selection from the editor's edit value foreach(object o in popupContainerEditor.EditValue as ArrayList) listBox.SetSelected(listBox.Items.IndexOf(o), true); } } } //Locates and retrieves a child ListBox control contained within the specified control private ListBox findChildListBox(Control parent) { foreach(Control control in parent.Controls) if (control is ListBox) return (ListBox)control; return null; } |
Visual Basic | ![]() |
---|---|
Imports DevExpress.XtraEditors.Controls Public Sub New() MyBase.New() InitializeComponent() '... popupContainerEdit1.EditValue = New ArrayList End Sub Private Sub popupContainerEdit1_QueryResultValue(ByVal sender As Object, _ ByVal e As QueryResultValueEventArgs) _ Handles popupContainerEdit1.QueryResultValue 'Get the list box displayed in the editor's dropdown Dim editor As PopupContainerEdit = CType(sender, PopupContainerEdit) Dim listBox As ListBox = findChildListBox(editor.Properties.PopupControl) 'Add selected items to the array specified by the editor's edit value Dim ar As ArrayList = CType(e.Value, ArrayList) ar.Clear() ar.AddRange(ListBox.SelectedItems) End Sub Private Sub popupContainerEdit1_QueryDisplayText(ByVal sender As Object, _ ByVal e As QueryDisplayTextEventArgs) _ Handles popupContainerEdit1.QueryDisplayText 'Return the string representing the selected item count Dim itemCount As Integer = CType(e.EditValue, ArrayList).Count e.DisplayText = itemCount.ToString() + " item(s) selected" End Sub Private Sub buttonOk_Click(ByVal sender As Object, ByVal e As System.EventArgs) _ Handles buttonOk.Click Dim button As Control = CType(sender, Control) 'Close the dropdown accepting the user's choice CType(button.Parent, PopupContainerControl).OwnerEdit.ClosePopup() End Sub Private Sub buttonCancel_Click(ByVal sender As Object, ByVal e As System.EventArgs) _ Handles buttonCancel.Click Dim button As Control = CType(sender, Control) 'Close the dropdown discarding the user's choice CType(button.Parent, PopupContainerControl).OwnerEdit.CancelPopup() End Sub Private Sub popupContainerEdit1_CloseUp(ByVal sender As Object, _ ByVal e As CloseUpEventArgs) Handles popupContainerEdit1.CloseUp 'Restore selected items if the user's choice should be discarded If Not e.AcceptValue Then Dim popupContainerEditor As PopupContainerEdit = CType(sender, PopupContainerEdit) 'Get the list box displayed in the dropdown of the popup container editor Dim listBox As ListBox = findChildListBox(popupContainerEditor.Properties.PopupControl) If Not listBox Is Nothing Then 'Clear existing selection listBox.ClearSelected() 'Restore selection from the editor's edit value For Each o As Object In CType(popupContainerEditor.EditValue, ArrayList) listBox.SetSelected(listBox.Items.IndexOf(o), True) Next End If End If End Sub 'Locates and retrieves a child ListBox control contained within the specified control Private Function findChildListBox(ByVal parent As Control) As ListBox For Each control As Control In parent.Controls If TypeOf control Is ListBox Then Return CType(control, ListBox) Next Return Nothing End Function |