组合框编辑器
组合框编辑器用于从固定或动态改变的列表中选择取值。 这些编辑器提供了一个编辑框和一个显示单列列表的下拉窗口。 这些编辑器从 PopupBaseEdit 类继承了公共的弹出窗口管理功能。 请参阅 下拉编辑器概述 主题获知其他信息。
XtraEditors 库提供了三种组合框编辑器:ComboBoxEdit、ImageComboBoxEdit 和 MRUEdit。
ComboBoxEdit 控件
ComboBoxEdit 控件在其下拉列表中显示了固定的列表项集。 不允许最终用户新增列表项 (除非您在代码中实现了此功能)。
在 ComboBoxEdit 控件中的每个列表项都是一个对象 (通常是字符串)。 要新增列表项,则需要把一个对象添加到 RepositoryItemComboBox.Items 集合中。 在下拉列表中显示的字符串是这些对象的文本版本,并且使用 ToString 方法能返回一个文本串。
下面的代码展示了如何把列表项添加到 ComboBoxEdit 控件中。
C# | ![]() |
---|---|
DevExpress.XtraEditors.Repository.RepositoryItemComboBox properties = comboBoxEdit1.Properties; properties.Items.AddRange(new string [] {"Barcelona", "Berlin", "Frankfurt", "Helsinki", "Kirkland", "London", "Madrid"}); //Select the first item comboBoxEdit1.SelectedIndex = 0; //Disable editing properties.TextEditStyle = DevExpress.XtraEditors.Controls.TextEditStyles.DisableTextEditor; |
Visual Basic | ![]() |
---|---|
Dim properties As DevExpress.XtraEditors.Repository.RepositoryItemComboBox = _ comboBoxEdit1.Properties properties.Items.AddRange(New string () {"Barcelona", "Berlin", "Frankfurt", _ "Helsinki", "Kirkland", "London", "Madrid"}) 'Select the first item comboBoxEdit1.SelectedIndex = 0 'Disable editing properties.TextEditStyle = DevExpress.XtraEditors.Controls.TextEditStyles.DisableTextEditor |
要编辑取值由列表定义的数据源字段,则可以使用列表项与这些取值相匹配的 ComboBoxEdit 控件。 但是在某些情况下,可能需要提供取值的特殊文本表示形式。 假设有一个存储整数值的“PaymentType”数据源字段,整数取值表示可能的付款方式 (0 - “现金”, 1 - “Visa”, 2 - “American Express”等)。 在这种情况下可能会使用 LookUpEdit 控件。 注意,仅当有一个特定的数据表时才使用 LookUpEdit 控件,在此数据表中列出了与编辑值对应的显示值。 如果不存在这样的数据表,则需要使用 ImageComboBoxEdit 控件来代替。
ComboBoxEdit 控件不对用户输入进行限制。 因此最终用户可以在编辑框中输入任何字符串,而不管列表项集。 要只允许选择列表项、禁用编辑框,则把 RepositoryItemButtonEdit.TextEditStyle 属性设置为 TextEditStyles.DisableTextEditor。
ImageComboBoxEdit 控件
ImageComboBoxEdit 控件在其下拉列表中显示一个列表项集。 此控件也支持在编辑框和下拉列表中显示列表项图像。 不支持编辑文本,因此只能通过从下拉列表中选择列表项来编辑取值。
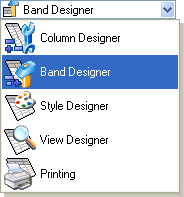
每个列表项都由一个 ImageComboBoxItem 对象表示,此对象提供了取值 (ComboBoxItem.Value)、说明 (ImageComboBoxItem.Description) 和图像属性 (ImageComboBoxItem.ImageIndex)。 在列表项被选中时,该项的值指定为编辑器的编辑值。 列表项的说明用于在屏幕上呈现该项。
ImageComboBoxEdit 支持列表项使用小图像或大图像。 图像由 RepositoryItemImageComboBox.SmallImages 和 RepositoryItemImageComboBox.LargeImages 属性指定。 如果为该编辑器提供了小图像和大图像,则在编辑框中的项显示有小图像,在列表框中的项显示有大图像。 只提供一个图像列表,则在下拉列表和编辑框中的同一项都显示相同的图像。 如果没有提供图像列表,则列表项仅由其说明呈现。
下面的示例代码展示了如何把列表项添加到 ImageComboBoxEdit 编辑器中。
C# | ![]() |
---|---|
using DevExpress.XtraEditors.Repository; RepositoryItemImageComboBox properties = imageComboBoxEdit1.Properties; //Specify lists with large and small images properties.LargeImages = imageList1; properties.SmallImages = imageList2; //Prevent updates while adding items properties.Items.BeginUpdate(); try { //Initialize each item with the display text, value and image index properties.Items.Add(new ImageComboBoxItem("Column Designer", 0, 0)); properties.Items.Add(new ImageComboBoxItem("Band Designer", 1, 1)); properties.Items.Add(new ImageComboBoxItem("Style Designer", 2, 2)); properties.Items.Add(new ImageComboBoxItem("View Designer", 3, 3)); properties.Items.Add(new ImageComboBoxItem("Printing", 4, 4)); } finally { properties.Items.EndUpdate(); } //Select the second item imageComboBoxEdit1.SelectedIndex = 1; |
Visual Basic | ![]() |
---|---|
Imports DevExpress.XtraEditors.Repository Dim properties As RepositoryItemImageComboBox = ImageComboBoxEdit1.Properties 'Specify lists with large and small images properties.LargeImages = ImageList1 properties.SmallImages = ImageList2 'Prevent updates while adding items properties.Items.BeginUpdate() Try 'Initialize each item with the display text, value and image index properties.Items.Add(New ImageComboBoxItem("Column Designer", 0, 0)) properties.Items.Add(New ImageComboBoxItem("Band Designer", 1, 1)) properties.Items.Add(New ImageComboBoxItem("Style Designer", 2, 2)) properties.Items.Add(New ImageComboBoxItem("View Designer", 3, 3)) properties.Items.Add(New ImageComboBoxItem("Printing", 4, 4)) Finally properties.Items.EndUpdate() End Try 'Select the second item ImageComboBoxEdit1.SelectedIndex = 1 |
MRUEdit 控件
使用 MRUEdit 控件允许最终用户从下拉列表中选择最近输入的取值。 MRUEdit 控件扩展了 ComboBoxEdit 控件的功能,允许最终用户把列表项添加到下拉列表中。 当最终用户输入一个新字符串并且把焦点移到其他控件或者按下 ENTER 键 (假设 RepositoryItemMRUEdit.ValidateOnEnterKey 属性值设置为 true) 时,此字符串被插入为下拉列表的顶部列表项。
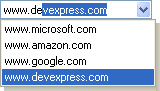
可以在下拉列表中显示的列表项的最大数目由 RepositoryItemMRUEdit.MaxItemCount 属性指定。 如果此属性值为 0,则不限制列表项的数目。 如果列表项的数目受限,则新增列表项可能会导致从列表中移除最近很少使用的列表项。
组合框编辑器的公共属性
所有组合框编辑器都支持自动完成功能。 这允许最终用户通过键入某项的起始字符,而从下拉列表中选择该项。 在编辑器中键入文本时,控件会查找以所键入字符开始的列表项,在下拉列表 (如果已打开) 中高亮显示该项,并且在编辑框中显示完整的列表项标题。 使用 RepositoryItemComboBox.AutoComplete 属性来启用或禁用自动完成功能。 RepositoryItemComboBox.CaseSensitiveSearch 属性指定自动完成是否区分大小写。 RepositoryItemPopupBaseAutoSearchEdit.ImmediatePopup 属性指定在用户开始键入时是否自动打开下拉列表。
当组合框获得焦点时,最终用户通过按下向上键和向下键来选择前/下一列表项,而无须打开下拉列表。 为了防止意外产生此行为,可以把 RepositoryItemComboBox.UseCtrlScroll 属性设置为 true。 在这种情况下,需要使用 CTRL+向上键 和 CTRL+向下键 组合键。
最终用户也可以通过双击编辑框遍历列表项来改变取值。 仅当 RepositoryItemComboBox.CycleOnDblClick 属性值设置为 true 并且 RepositoryItemPopupBase.ShowDropDown 属性值设置为 ShowDropDown.Never 时可用。
下拉列表的高度由 RepositoryItemComboBox.DropDownRows 属性控制。 要指定列表项的高度,则使用 RepositoryItemComboBox.DropDownItemHeight 属性。 如果需要使用 ComboBoxEdit.DrawItem 事件自定义绘制下拉列表,则这是有用的。